How to Optimize Web Application Performance with Angular Hydration
Angular hydration is a technique that enhances web application performance by optimizing how Angular applications are rendered and updated. By ensuring that the server-rendered content and the client-side application stay in sync, hydration reduces the time it takes for the application to become interactive. This leads to faster load times and a more responsive user experience. Understanding how hydration works can help developers make informed decisions about their rendering strategies and improve overall application efficiency.
To leverage Angular hydration effectively, it's important to implement best practices such as optimizing server-side rendering (SSR) and minimizing client-side JavaScript processing. By carefully managing the transition between server-rendered content and client-side interactivity, developers can ensure a smoother user experience and enhance application performance. Hydration not only improves load times but also contributes to better SEO and overall user satisfaction.
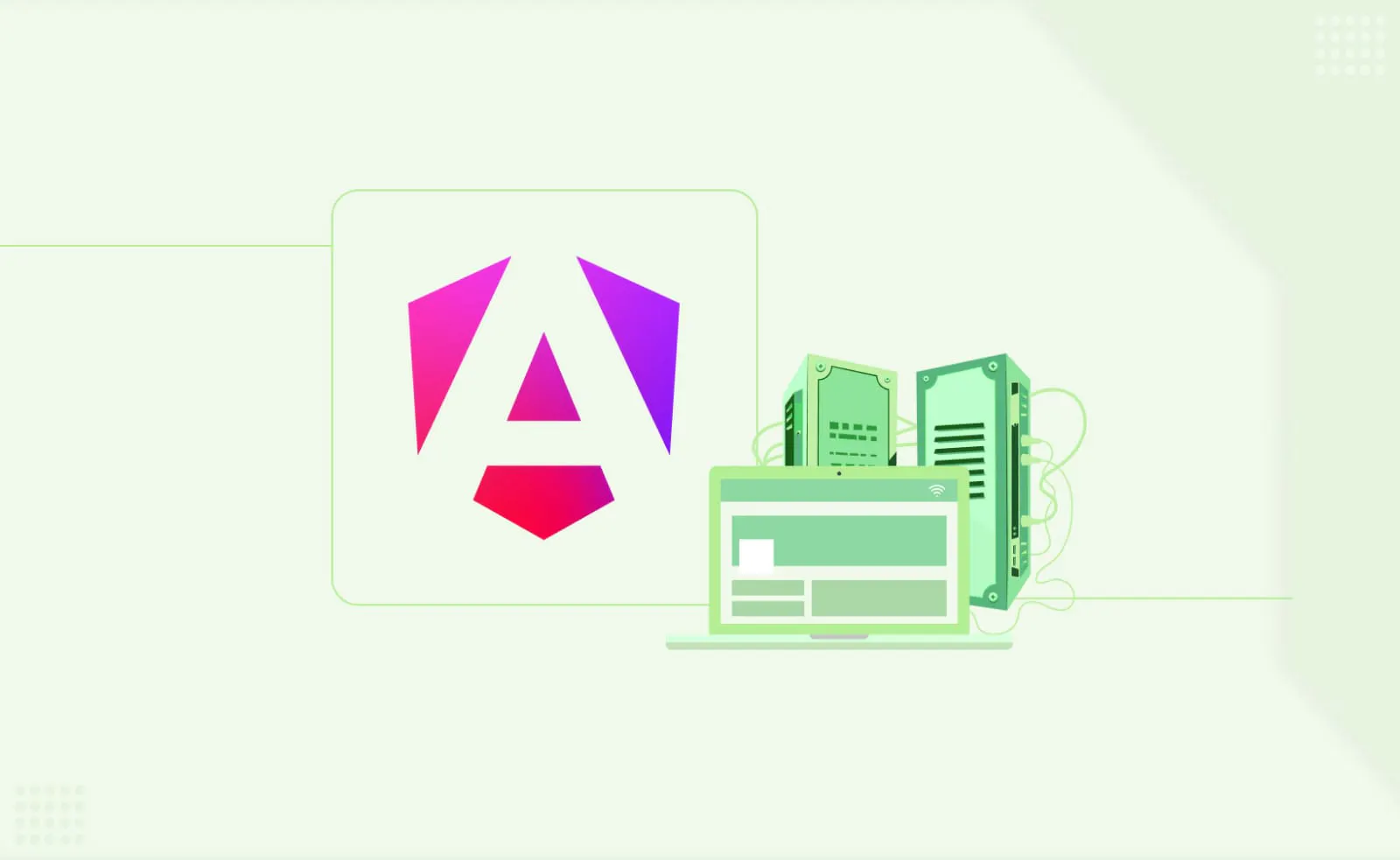
Angular Hydration plays a pivotal role in server-side rendering (SSR) by boosting the performance and interactivity of Angular applications. By grasping how hydration operates and its advantages, you can enhance both the performance and user experience of your Angular apps. This guide will delve into the concept of Angular Hydration, its role in the SSR workflow, various rehydration techniques, alternatives to rehydration, and the benefits it offers.
Understanding Angular Hydration is essential for optimizing your applications. It involves synchronizing server-rendered content with client-side interactions to achieve smoother transitions and faster load times. Through this guide, you'll learn how to effectively integrate hydration into your SSR strategy to improve overall application efficiency and user satisfaction.
Introduction to Angular Hydration
In Angular, hydration involves the transition where client-side JavaScript takes control of the HTML content rendered by the server, turning the static page into an interactive one. When Angular Universal is used for server-side rendering (SSR), the server generates and delivers the initial HTML to the client. Hydration then occurs on the client side, where Angular initializes, adding dynamic features and interactivity to the page.
How Hydration Works in Angular
1. Server-Side Rendering (SSR):
- The server handles the request for a specific page.
- Angular Universal processes and renders the requested page on the server, producing the HTML content.
- The server then sends this fully rendered HTML back to the client's browser.
2. Initial HTML Load:
- The browser receives and displays the HTML content immediately.
- Users see a complete page without having to wait for the client-side JavaScript to load and execute.
3. Client-Side Hydration:
- Angular initializes and bootstraps the application on the client side.
- Angular compares the HTML rendered by the server with the state of the client-side application.
- Interactive elements and event listeners are attached, making the page dynamic and fully interactive.
Example Scenario
Home Page (/):
- The server processes and generates the HTML for the HomeComponent.
- The browser immediately renders and displays the HomeComponent's content.
- On the client side, Angular initializes, allowing interactive features like button clicks and form submissions to function.
About Page (/about):
- The server handles the request and creates the HTML for the AboutComponent.
- The browser promptly shows the AboutComponent's content.
- Angular then bootstraps on the client side, enabling the page's interactive elements to work properly.
Benefits of Angular Hydration
- Improved Performance:
Hydration enables the browser to quickly present content, minimizing the wait time for users to see the fully rendered page. This results in faster perceived load times and enhanced performance, particularly beneficial for users on slower networks or devices.
- Enhanced SEO:
Server-rendered HTML content is easier for search engines to crawl and index, which boosts your application's SEO. With hydration, interactive elements are integrated after the initial HTML content is loaded, maintaining SEO advantages while enabling dynamic functionality.
- Better User Experience:
Users enjoy a smooth transition from static content to an interactive application. The initial HTML load provides immediate and complete content visibility, and data hydration ensures that interactive features are added without noticeable delays.
Types of Rehydration
- Partial Rehydration:
Partial rehydration allows developers to add interactive features only to specific sections of a website while keeping the rest of the content static. This technique reduces the amount of JavaScript required for hydration, leading to improved load times and overall performance.
- Progressive Rehydration:
Progressive rehydration gradually initializes different functionalities of a server-rendered application over time, rather than all at once. This approach ensures that the most critical elements of the page become interactive first, with less essential components being hydrated subsequently.
- Trisomorphic Rendering:
Trisomorphic rendering employs streaming server-side rendering for initial or non-JS navigations, and then uses a service worker framework to handle HTML rendering for subsequent navigations after installation. This method blends the advantages of server-side rendering, client-side rendering, and service workers to deliver a highly efficient and interactive application.
Setting Up Angular Hydration
Step 1: Set Up Your Angular Application
If you haven't yet set up an Angular application, you can create one by using the Angular CLI:
ng new angular-hydration-app cd angular-hydration-app
Step 2: Integrate Angular Universal
Incorporate Angular Universal into your project by executing the command below:
ng add @nguniversal/express-engine
Step 3: Set Up Server-Side Rendering Configuration
Verify that your server.ts
file is properly configured to manage server-side rendering (SSR). This setup
is typically handled automatically when you integrate Angular Universal.
Step 4: Develop Components and Configure Routing
Create the necessary components and establish routing as required. For instance:
ng generate component home --module=app.module ng generate component about --module=app.module
Update your app-routing.module.ts:
import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; import { HomeComponent } from './home/home.component'; import { AboutComponent } from './about/about.component'; const routes: Routes = [ { path: '', component: HomeComponent }, { path: 'about', component: AboutComponent }, ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { }
Update your app.component.html:
Step 5: Build and Serve the Application
Build the application for SSR:
npm run build:ssr
Serve the application code:
npm run serve:ssr
Visit http://localhost:4000 to view your Angular application with server-side rendering and hydration in action.
Real-World Example
E-commerce Website Scenario
Imagine an e-commerce site developed using Angular. This site includes various product pages, a shopping cart feature, and a checkout process.
Without Hydration
- The server delivers static HTML to the client.
- Users must wait for client-side JavaScript to load and execute before the page becomes interactive.
- Initial load times can be slow, particularly on slower networks or devices.
With Hydration
- The server sends pre-rendered HTML to the client.
- Content is visible immediately as the HTML loads.
- Angular initializes on the client side, enabling interactive features like adding products to the cart and proceeding to checkout.
- Initial load times are faster, leading to a better user experience.
Benefits of This Scenario
- Faster Load Time: Users can view and interact with content right away.
- Better SEO: Pre-rendered HTML is easily indexed by search engines.
- Enhanced User Experience: Smooth transition from static content to a fully interactive application.
Alternatives to Rehydration
Resumability
Resumability is a technique that eliminates the need for rehydration and its associated overhead. Instead of repeating the server's work, resumability generates the required JavaScript only when needed in response to user interactions. This method can enhance performance by reducing the amount of JavaScript that needs to be executed during the initial page load.
Conclusion
In this guide, we’ve delved into Angular Hydration, its function in the server-side rendering process, and the advantages it offers for Angular applications. We covered various rehydration techniques, including partial rehydration, progressive rehydration, and trisomorphic rendering, as well as alternatives like resumability. By pre-rendering HTML on the server and initializing Angular on the client side, hydration enhances performance, SEO, and user experience.
We also walked through the steps to implement Angular Hydration and provided a practical example to demonstrate its benefits. By incorporating Angular Hydration, you can significantly improve the performance and user experience of your Angular applications. Experiment with hydration techniques in your projects to experience the benefits firsthand.
Feel free to apply these insights to optimize your Angular applications and observe the positive impact on both performance and user satisfaction. Exploring and implementing hydration can lead to a more efficient and engaging web application.
Please complete your information below to login.