How to Manage State in Angular Applications Using NgRx
Discover how to effectively manage state in your Angular applications with NgRx. This comprehensive guide will walk you through the core concepts of state management, including actions, reducers, and effects, and show you how to implement them to keep your application's state predictable and consistent.
Learn practical techniques for setting up NgRx in your project, handling complex state interactions, and leveraging powerful tools like selectors and the store. By the end of this guide, you'll be equipped to manage state efficiently and scale your Angular applications with confidence.
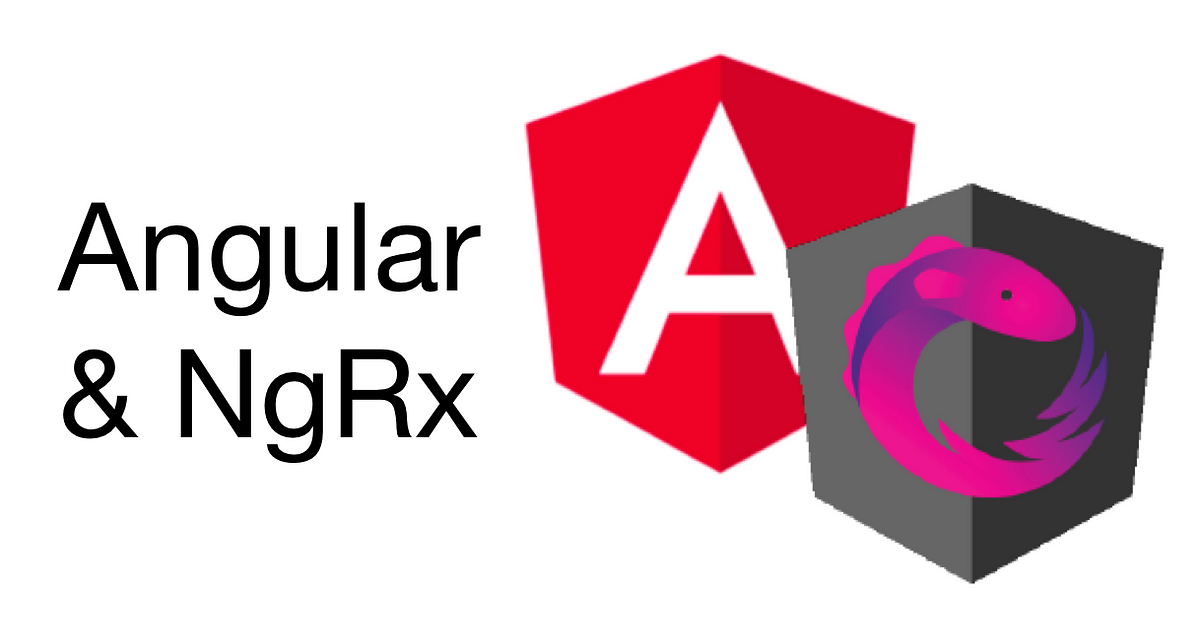
State management is essential for developing complex applications, particularly in Angular where components frequently need to share and synchronize data. Although Angular offers its own state management solutions, such as services and component interactions, these can become unwieldy as the application scales and becomes more intricate. This is where NgRx comes in, providing a powerful state management solution inspired by Redux.
NgRx simplifies state management by introducing a structured approach to handling state, actions, and side effects. With its well-defined patterns and tools, NgRx helps maintain a clean and scalable architecture, making it easier to manage and debug your Angular application's state as it grows.
What is Angular State Management with NgRx?
NgRx is a state management library specifically designed for Angular applications, drawing heavy inspiration from Redux, a state container for JavaScript applications. It adheres to the Redux principles of unidirectional data flow and immutable state, offering a reliable and scalable way to handle application state.
Why Use NgRx for State Management?
Predictability and Debuggability
NgRx ensures a single source of truth for your application's state, making it simpler to trace and comprehend how data evolves over time.
Scalability
NgRx excels in managing state as your application expands, thanks to its centralized state store and methodical approach to managing asynchronous actions.
Tooling and DevTools
NgRx offers robust developer tools, such as NgRx DevTools, which allow you to observe and monitor state changes in real-time, aiding in debugging and enhancing performance.
Code Organization
NgRx promotes a well-organized and disciplined method for managing your application's logic, clearly separating tasks like data retrieval, state updates, and UI changes.
Core Concepts of NgRx store
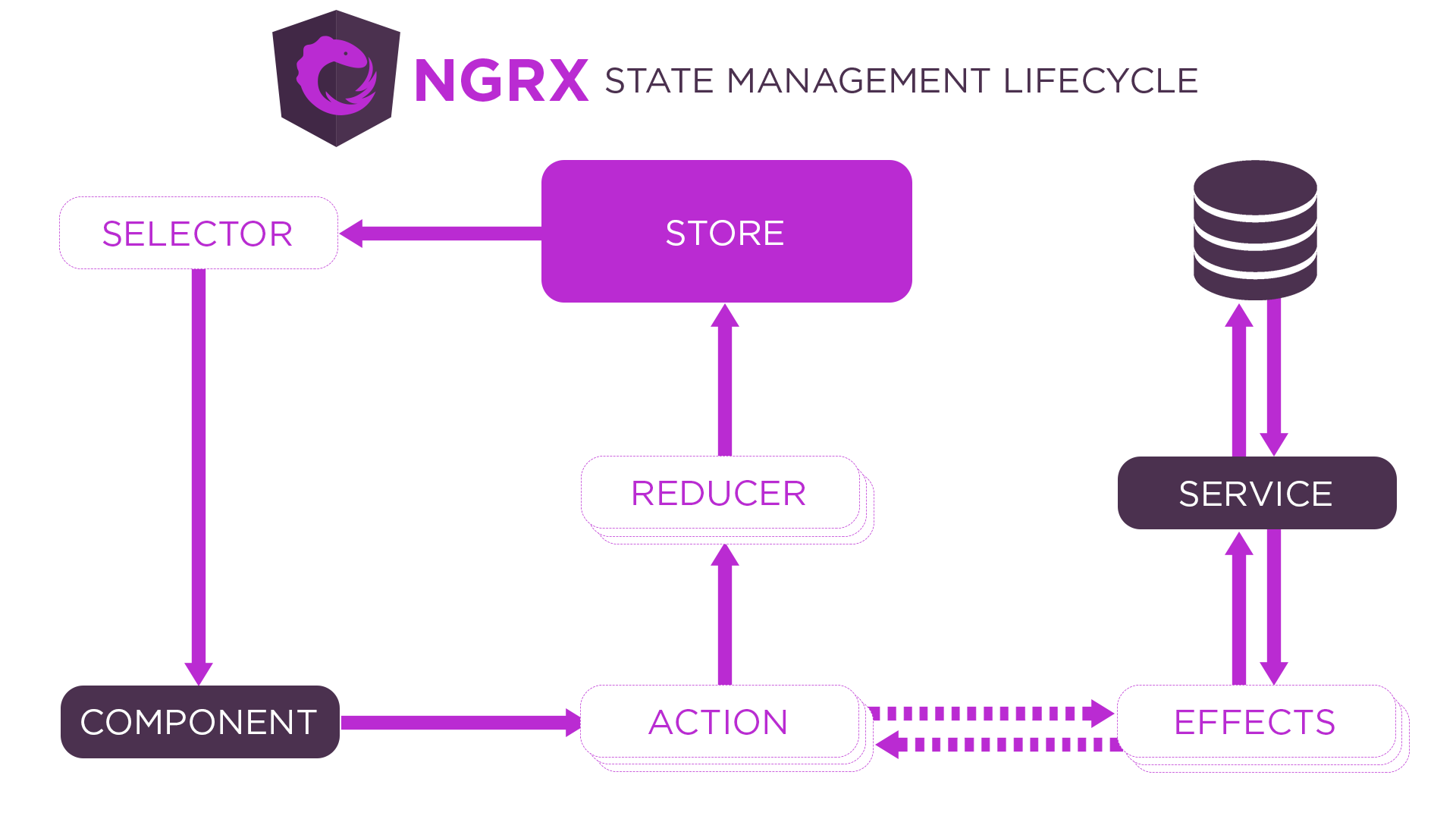
Store
The core component of NgRx is the Store, which maintains the application's state. It is an immutable entity that can only be changed by dispatching actions. Components interact with the store by using selectors to retrieve specific segments of the state.
Actions
Actions are simple objects that signify distinct events or intentions, representing occurrences within the application. These actions are sent to the store, which then updates the state through reducers.
Reducers
Reducers are pure functions tasked with taking the current state and an action to produce a new state. They are the sole mechanism for altering state in NgRx, ensuring that changes are predictable and traceable.
Selectors
Selectores are pure functions designed to access, derive, and compose parts of the state from the store. They offer a memoized method to retrieve specific state portions without recalculating unless there is a change in the underlying state.
Effects
Effects handle side effects such as asynchronous tasks and data interactions (e.g., HTTP requests). They listen for actions dispatched to the store, execute necessary logic (like data retrieval), and then dispatch additional actions with the outcomes.
Creating Actions, Reducers, States, and Selectors
Creating Actions, Reducers, State, and Selectors in Angular with NgRx
Managing application state in Angular using NgRx involves several key components. Let’s explore each of these in detail:
Actions
In NgRx, actions represent events or intents that indicate something has occurred within the application. They facilitate communication regarding state changes, transitions, and interactions in the Angular application's state management system.
Define Action Types
Action types are constants that specify the nature of the action being dispatched. They are usually defined in a separate file to maintain consistency and prevent errors from typos.
// item.actions.ts import { createAction, props } from '@ngrx/store'; import { Item } from './item.model'; // Replace with your model if applicable export enum ItemActionTypes { LoadItems = '[Item] Load Items', LoadItemsSuccess = '[Item] Load Items Success', LoadItemsFailure = '[Item] Load Items Failure', } export const loadItems = createAction( ItemActionTypes.LoadItems ); export const loadItemsSuccess = createAction( ItemActionTypes.LoadItemsSuccess, props<{ items: Item[] }>() ); export const loadItemsFailure = createAction( ItemActionTypes.LoadItemsFailure, props<{ error: any }>() );
In this Example:
The loadItems
action does not carry any payload.
The loadItemsSuccess
action contains an array of items as its payload.
The loadItemsFailure
action includes an error object as its payload.
Using Actions
Actions can be dispatched from various parts of your application, including components, services, effects, and other sections of your codebase.
// item-list.component.ts import { Component, OnInit } from '@angular/core'; import { Store } from '@ngrx/store'; import { Observable } from 'rxjs'; import { AppState } from './app.state'; // Replace with your state interface import { Item } from './item.model'; // Replace with your model if applicable import { loadItems } from './item.actions'; // Import your actions @Component({ selector: 'app-item-list', templateUrl: './item-list.component.html', styleUrls: ['./item-list.component.css'] }) export class ItemListComponent implements OnInit { items$: Observable- ; constructor(private store: Store
) { } ngOnInit(): void { this.items$ = this.store.select(state => state.items); // Example selector, adjust as per your state structure this.store.dispatch(loadItems()); // Dispatch the loadItems action } }
Reducers
Reducers define how the state of the application is updated in response to actions dispatched to the store. Each reducer function receives the current state from the store and an action, and returns the updated state.
// item.reducer.ts import { createReducer, on } from '@ngrx/store'; import { Item } from './item.model'; // Replace with your model if applicable import { loadItemsSuccess, loadItemsFailure } from './item.actions'; // Import your actions export interface ItemState { items: Item[]; loading: boolean; error: any; } export const initialState: ItemState = { items: [], loading: false, error: null }; export const itemReducer = createReducer( initialState, on(loadItemsSuccess, (state, { items }) => ({ ...state, items, loading: false, error: null })), on(loadItemsFailure, (state, { error }) => ({ ...state, loading: false, error })) );
State
The application state is handled as an object within the NgRx store module.
This state consists of multiple slices, each governed by its own reducer.
State encompasses both data and the user interface (UI) conditions of an application. Here’s how you define the overall application state:
// app.state.ts import { ItemState } from './item.reducer'; // Import your specific state interfaces export interface AppState { items: ItemState; }
Selectors
Selectors are pure functions that receive the entire application state as input and return a specific slice of that state. They are utilized to encapsulate the logic for accessing particular segments of the Angular application state from the store:
// item.selectors.ts import { createSelector, createFeatureSelector } from '@ngrx/store'; import { AppState } from './app.state'; // Replace with your state interface import { ItemState } from './item.reducer'; // Replace with your specific state interface export const selectItemState = createFeatureSelector('items'); export const selectItems = createSelector( selectItemState, (state: ItemState) => state.items ); export const selectLoading = createSelector( selectItemState, (state: ItemState) => state.loading ); export const selectError = createSelector( selectItemState, (state: ItemState) => state.error );
Registering Modules in AppModule
To complete the setup, you need to register your reducers and effects (if any) in the AppModule. This step integrates them with NgRx's store:
// app.module.ts import { NgModule } from '@angular/core'; import { BrowserModule } from '@angular/platform-browser'; import { StoreModule } from '@ngrx/store'; import { EffectsModule } from '@ngrx/effects'; import { HttpClientModule } from '@angular/common/http'; import { AppComponent } from './app.component'; import { itemReducer } from './item.reducer'; // Import your reducers import { ItemEffects } from './item.effects'; // Import your effects if applicable @NgModule({ declarations: [ AppComponent ], imports: [ BrowserModule, HttpClientModule, StoreModule.forRoot({ items: itemReducer }), // Register your reducers EffectsModule.forRoot([ItemEffects]) // Register your effects if applicable ], providers: [], bootstrap: [AppComponent] }) export class AppModule { }
Getting Started with NgRx
1. Installation
To incorporate NgRx into your Angular project, begin by installing the required packages:
ng add @ngrx/store
This command installs NgRx along with essential packages such as @ngrx/effects
,
@ngrx/store-devtools
, and @ngrx/entity
.
Alternatively, you can also install NgRx using npm with the following command:
npm install @ngrx/store --save
2. Setting Up the Store
Define your application's state interface, and create actions, reducers, effects, and selectors according to your application's needs.
3. Dispatching Actions
Dispatch actions from your Angular components or services to initiate state changes:
this.store.dispatch(loadItems()); // E
4. Selecting State
Use selectors to retrieve slices of state from the store
this.items$ = this.store.select(selectItems); // Example selector usage
5. Handling Effects
Implement effects to manage side effects such network requests such as HTTP requests or other asynchronous operations:
@Effect() loadItems$ = this.actions$.pipe( ofType(loadItems), mergeMap(() => this.itemService.getItems().pipe( map(items => loadItemsSuccess({ items })), catchError(error => of(loadItemsFailure({ error }))) ) ) );
Pros and Cons of NGRX store
NgRx Store
NgRx Store is a state management library designed for Angular applications, drawing inspiration from Redux in the React ecosystem.
It offers a single state tree and enforces unidirectional data flow, which can be particularly useful for handling complex application states. Here are some advantages and disadvantages of using NgRx Store:
Pros:
Predictable State Management
With a single source of truth, the state becomes more predictable and easier to debug. Each action produces a new state, making data flow clearer and more manageable.
Time-Travel Debugging
NgRx Store's time-travel debugging feature allows developers to rewind and replay actions, simplifying the process of identifying and resolving bugs.
Decoupled Architecture
The separation of state management from UI components results in a more modular and testable codebase, which enhances maintainability and scalability.
Single Source of Truth
All application state is contained within a single object, streamlining state management, especially in large applications with complex interactions.
Middleware Integration
NgRx offers robust middleware options, such as effects, which handle side effects (e.g., API requests) in a clean and organized manner.
Community and Ecosystem
NgRx is widely used within the Angular community, offering a strong ecosystem of tools, extensions, and support.
Cons:
Learning Curve
Developers new to reactive programming or state management libraries like Redux might find the concepts of reducers, actions, effects, and selectors challenging to grasp.
Boilerplate Code
NgRx necessitates a considerable amount of boilerplate code, which can be daunting, particularly for small projects or straightforward state management scenarios.
Complexity
For smaller applications, NgRx might add unnecessary complexity. The setup and maintenance of the store might outweigh the benefits for simpler needs.
Performance Overhead
Although NgRx performs well, improper use of selectors or frequent state updates can lead to performance issues. Efficient use of selectors is essential to prevent unnecessary re-renders.
Steep Integration
Integrating NgRx into an existing project can be challenging, often requiring substantial refactoring and a re-evaluation of the current state management strategy.
Opinionated Structure
NgRx imposes a specific structure and flow, which might not fit every project. This rigidity can be a drawback if a more flexible approach is needed.
In summary, while NgRx Store provides powerful state management features suitable for complex applications, it involves a learning curve and potential added complexity. Assessing the project's requirements and the team's familiarity with the concepts is crucial when considering NgRx Store.
Conclusion
NgRx offers a robust and scalable solution for managing state in Angular applications, delivering predictability, scalability, and effective debugging tools. By adopting the Redux pattern and utilizing its ecosystem, NgRx assists developers in creating maintainable and high-performance applications.
If you're launching a new Angular project or updating an existing one to manage complex state more effectively, think about incorporating NgRx into your architecture for a more organized and maintainable codebase.
Please complete your information below to login.