How to Develop Modular Plugins in Angular: A Comprehensive Guide
Discover the process of building modular plugins in Angular with this in-depth guide. Learn how to create plugins that enhance your Angular applications by offering reusable, self-contained components.
This guide will walk you through the essential steps, ensuring you can efficiently develop and integrate modular plugins within your Angular projects.
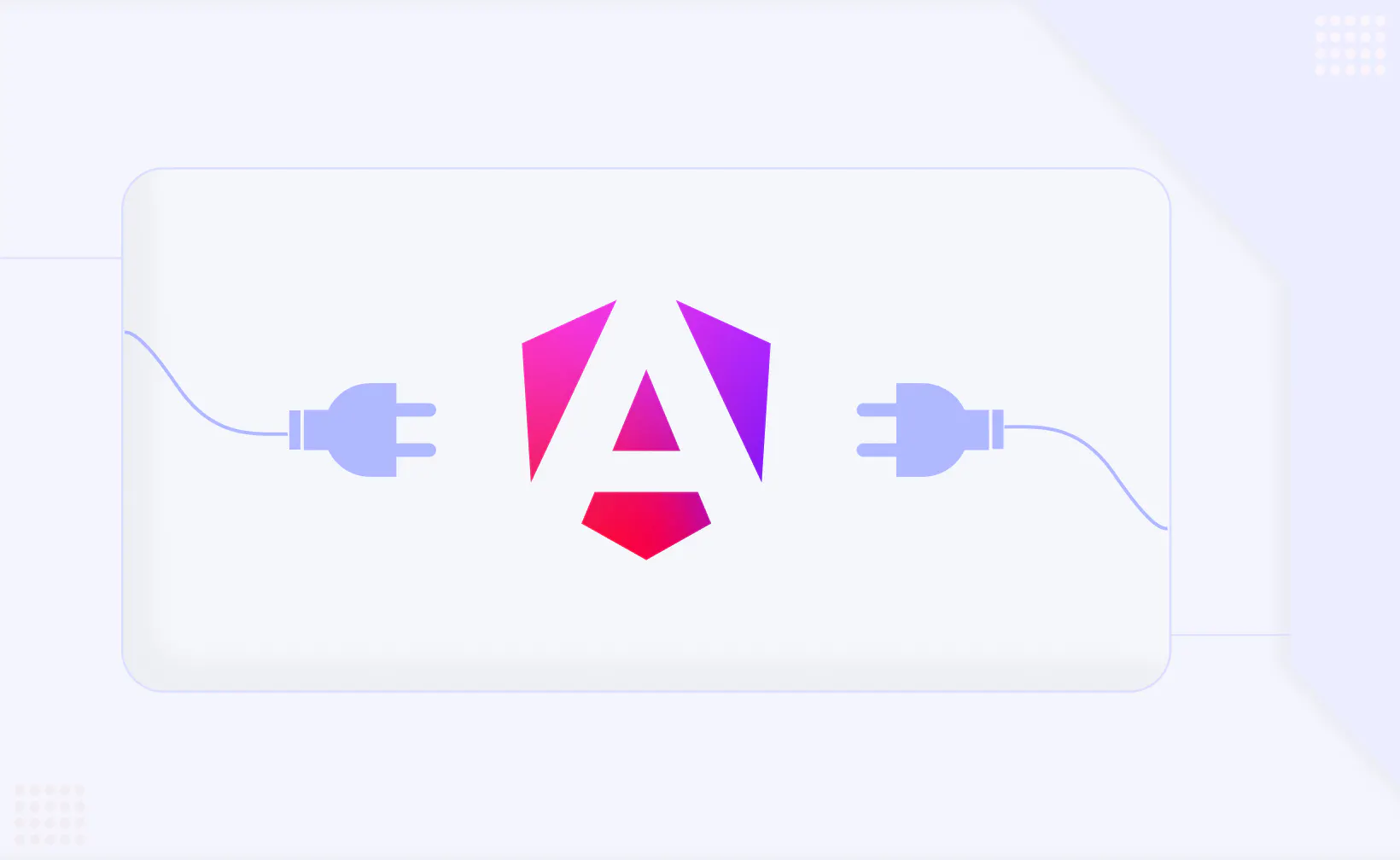
Understanding Angular Plugins
The landscape of web development is evolving, and Angular is driving many of these advancements. Angular is known for delivering powerful, efficient, and highly scalable applications. However, one challenge in application development is the need for a plugin architecture in Angular that can expand the application's features and functionalities without compromising its maintainability.
What is a plugin in Angular?
In Angular, a plugin is a reusable code module designed to enhance the capabilities of your Angular application. These plugins can introduce a range of features and functions, helping you to avoid redundant coding and concentrate on developing the core aspects of your web application.
Below are some key features of Angular plugins:
Modularity: Plugins are independent units that include components, directives, services, and pipes. This modular approach facilitates code reusability and simplifies maintenance.
Extensibility: Angular plugins are created to be flexible and adaptable. You can configure them to integrate smoothly with your specific application needs.
Third-Party or Custom Development: You have the option to use pre-existing plugins from various sources or develop your own custom plugins to meet particular requirements within your Angular application.
Benefits of using plugins in Angular applications
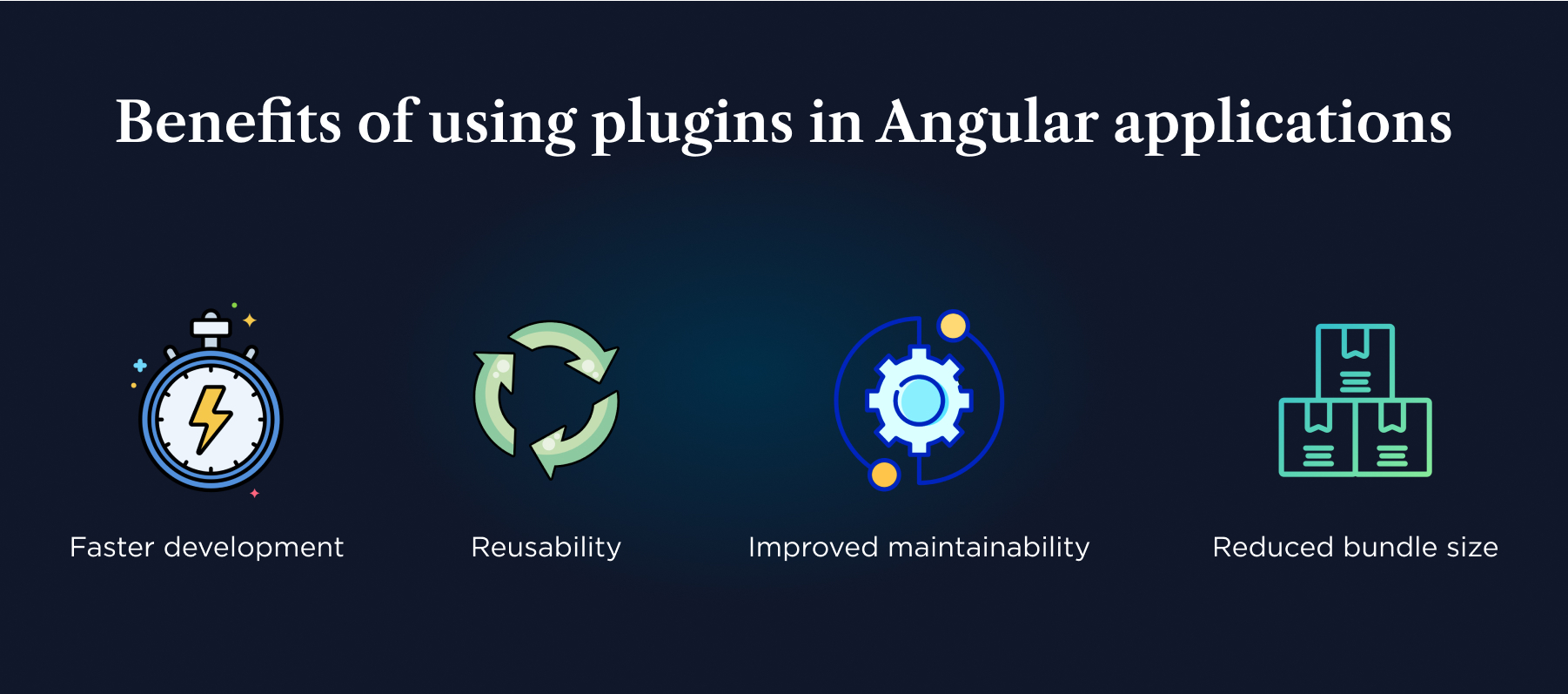
Integrating plugins into your Angular applications offers several benefits:
Faster Development: Plugins deliver pre-built functionalities, which saves you time and effort compared to creating everything from scratch. You can access plugins for various common features such as charts, data tables, drag-and-drop capabilities, and more.
Reusability: Many plugins are thoroughly tested and maintained by a community of developers. This means you can rely on stable code and benefit from regular updates and bug fixes.
Improved Maintainability: Using plugins for specific features allows you to keep your core application streamlined, enhancing the overall maintainability of your codebase. This approach makes it easier to understand, modify, and debug your application in the future.
Optimized Bundle Size: By utilizing only the necessary features from a plugin, you can help reduce the size of your application's bundle. This can result in faster loading times for your users.
Setting Up the Plugin Architecture in Angular

Setting up the plugin architecture might appear complex, but we've simplified the process with the following steps:
Step 1: Define the Plugin Interface
Start by creating an interface that specifies the fundamental structure of a plugin. This interface should include methods for initialization, configuration, and supplying components or services.
TypeScript import { Injectable } from '@angular/core'; export interface Plugin { id: string; name: string; version: string; initialize(): void; configure(config: any): void; getComponents(): any[]; getServices(): any[]; }
Step 2: Develop a Plugin Manager Service
Create a service responsible for managing the lifecycle of plugins. This service will manage tasks such as loading, registering, and initializing the plugins.
TypeScript import { Injectable } from '@angular/core'; import { Plugin } from './plugin.interface'; @Injectable({ providedIn: 'root' }) export class PluginManagerService { private plugins: Plugin[] = []; loadPlugin(plugin: Plugin) { this.plugins.push(plugin); plugin.initialize(); } getPlugins(): Plugin[] { return this.plugins; } }
Step 3: Develop a Plugin Module
Build a foundational plugin module that other plugins can extend. This module should offer shared functionalities or interfaces that other plugins can utilize.
TypeScript import { NgModule } from '@angular/core'; @NgModule({ // Shared components or services }) export class BasePluginModule {}
Step 4: Build Plugins
Develop separate plugin modules, with each one implementing the Plugin interface.
TypeScript import { NgModule } from '@angular/core'; import { Plugin } from './plugin.interface'; import { BasePluginModule } from './base-plugin.module'; @NgModule({ imports: [BasePluginModule], // Plugin-specific components and services }) export class MyPluginModule implements Plugin { id = 'my-plugin'; name = 'My Plugin'; version = '1.0.0'; initialize() { // Plugin initialization logic } configure(config: any) { // Plugin configuration logic } getComponents() { // Return plugin components } getServices() { // Return plugin services } }
Step 5: Load and Register Plugins
In your main application, use the PluginManagerService to load and register the plugins.
TypeScript import { Component, OnInit } from '@angular/core'; import { PluginManagerService } from './plugin-manager.service'; import { MyPluginModule } from './my-plugin.module'; @Component({ selector: 'app-root', templateUrl: './app.component.html', styleUrls: ['./app.component.css'] }) export class AppComponent implements OnInit { constructor(private pluginManager: PluginManagerService) {} ngOnInit() { this.pluginManager.loadPlugin(new MyPluginModule()); // Load other plugins } }
Building and Integrating the Plugin
Implementing lazy-loaded modules in a plugin
Create a Feature Module:
Develop a distinct NgModule dedicated to the plugin's features.
This module should include components, directives, pipes, and services that are specific to the plugin.
TypeScript import { NgModule } from '@angular/core'; import { CommonModule } from '@angular/common'; import { RouterModule } from '@angular/router'; import { PluginComponent } from './plugin.component'; @NgModule({ imports: [ CommonModule, RouterModule.forChild([ { path: '', component: PluginComponent } ]) ], declarations: [PluginComponent], }) export class PluginModule {}
Configure Lazy Loading:
In the main application's routing module, use the loadChildren
property to set up the route for lazy
loading.
TypeScript import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'plugin', loadChildren: () => import('./plugins/my-plugin/plugin.module').then(m => m.PluginModule) } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule {}
Integrating plugins with the core: dependency management and isolation
Dependency Management:
Shared Dependencies:
If both the core application and plugins use the same dependencies, ensure that the versions are consistent to prevent conflicts. Utilize a package manager like npm or yarn to handle these shared dependencies.
Plugin-Specific Dependencies:
Keep plugin dependencies separate to avoid issues with the core application. For services that are used across the
application, use providedIn: 'root'
carefully to manage their scope.
Dependency Injection:
Plugin-Specific Services:
Declare services within the plugin module to confine their scope. Use providedIn: 'any'
to enable
dynamic injection of services.
Shared Services:
To share services between the core application and plugins, consider creating a shared library or module.
Isolation:
Plugin-Specific Styling:
Employ CSS modules or scoped styles to prevent style conflicts.
Avoid Naming Conflicts:
Use unique names for components, directives, pipes, and services to avoid naming collisions.
Consider a Plugin Registry:
Manage plugin metadata and dependencies from a central registry.
TypeScript // Core module import { NgModule } from '@angular/core'; import { SharedService } from './shared.service'; @NgModule({ providers: [SharedService] }) export class CoreModule {} // Plugin module import { NgModule } from '@angular/core'; import { SharedService } from '../core/shared.service'; @NgModule({ providers: [ { provide: SharedService, useExisting: SharedService } // Inject shared service ] }) export class PluginModule {}
Creating a Dynamic Plugin Loader:
Dynamic component rendering and event handling within plugins can be achieved using Angular's
ComponentFactoryResolver
. This tool enables the creation and insertion of components into the DOM on
the fly.
TypeScript import { ComponentFactoryResolver, Injector, ComponentRef } from '@angular/core'; @Component({ // ... }) export class HostComponent { constructor(private componentFactoryResolver: ComponentFactoryResolver, private injector: Injector) {} loadComponent(componentType: any) { const componentFactory = this.componentFactoryResolver.resolveComponentFactory(componentType); const componentRef: ComponentRef= this.componentFactoryResolver.createEmbeddedView(componentFactory); // Append the component to the host element } }
Event Handling:
Output Properties:
Plugins can trigger events using Angular's Output
decorator.
Custom Event Emitter:
Develop a custom event emitter service to facilitate global event communication.
Observables:
Utilize Observables to manage asynchronous communication.
TypeScript import { Output, EventEmitter } from '@angular/core'; export class PluginComponent { @Output() pluginEvent = new EventEmitter(); emitEvent(data: any) { this.pluginEvent.emit(data); } }
Building a Plugin Loader Module:
Create a plugin loader module to handle the management of plugins within the application.
Plugin Interface:
Establish a standard interface that all plugins must follow.
TypeScript export interface Plugin { id: string; name: string; component: any; // Component to be loaded config?: any; // Optional configuration }
Plugin Loader Service
TypeScript import { Injectable } from '@angular/core'; import { ComponentFactoryResolver, Injector } from '@angular/core'; import { Plugin } from './plugin.interface'; @Injectable({ providedIn: 'root' }) export class PluginLoaderService { private plugins: Plugin[] = []; constructor(private componentFactoryResolver: ComponentFactoryResolver, private injector: Injector) {} loadPlugin(plugin: Plugin) { this.plugins.push(plugin); // Load the component dynamically } unloadPlugin(pluginId: string) { // Remove the plugin from the list and destroy the component } }
Plugin Management:
Store Plugin Metadata:
Keep plugin metadata such as ID, name, and status in a centralized storage solution, such as local storage or a database.
Plugin Discovery:
Implement methods for discovering plugins, which may include using a file system or accessing a remote repository.
Plugin Lifecycle Management:
Manage the plugin lifecycle by handling tasks such as loading, unloading, and updating plugins.
Implementing Dependency Injection in Plugins
Integrating plugins into a core Angular application and managing dependencies effectively involves meticulous planning. The objective is to allow plugins to access required services without causing conflicts and to maintain proper isolation.
Implementing a plugin architecture with dependency injection
Define a Plugin Interface
TypeScript import { Injector } from '@angular/core'; export interface Plugin { id: string; name: string; initialize(injector: Injector): void; // ... other plugin methods }
Create a Plugin Loader Service
TypeScript Here is the TypeScript code with extra spaces removed: ```typescript import { Injectable, Injector } from '@angular/core'; import { Plugin } from './plugin.interface'; @Injectable({ providedIn: 'root' }) export class PluginLoaderService { private plugins: Plugin[] = []; constructor(private injector: Injector) {} loadPlugin(plugin: Plugin) { plugin.initialize(this.injector); this.plugins.push(plugin); } // ... other plugin management methods } ```
Inject Dependencies in Plugins
TypeScript import { Injectable, Injector } from '@angular/core'; import { Plugin } from './plugin.interface'; import { SomeCoreService } from '../core/some-core.service'; @Injectable() export class MyPlugin implements Plugin { constructor(private coreService: SomeCoreService) {} initialize(injector: Injector) { // Access core service and plugin logic } }
Managing dependencies in a plugin to the Angular core
Shared Dependencies:
For services used throughout the application, apply providedIn: 'root'
to ensure they are accessible.
Maintain consistent versions for shared dependencies to prevent conflicts.
Plugin-Specific Dependencies:
Declare dependencies within the plugin module itself.
Avoid using providedIn: 'root'
for services that are specific to the plugin.
Dependency Injection:
Inject the necessary dependencies directly into the plugin's constructor.
Utilize the provided Injector
in the initialize method to access any additional dependencies if
required.
Isolation:
Adopt clear naming conventions for plugin components, services, and directives to avoid confusion.
Use CSS modules or scoped styles to avoid conflicts with existing styles.
Refrain from directly manipulating the core application's DOM.
TypeScript // Core module import { NgModule } from '@angular/core'; import { SharedService } from './shared.service'; @NgModule({ providers: [SharedService] }) export class CoreModule {} // Plugin module import { NgModule } from '@angular/core'; import { SharedService } from '../core/shared.service'; import { PluginService } from './plugin.service'; @NgModule({ providers: [PluginService] // Plugin-specific service }) export class PluginModule {}
Using the Plugin in an Angular Application
Consuming a plugin in an Angular application
Importing the Plugin:
Direct Import: If the plugin is a local library, import it directly into your application's module.
TypeScript import { PluginModule } from 'path/to/plugin'; @NgModule({ imports: [ // ... PluginModule ], // ... }) export class AppModule {}
Dynamic Import:
If the plugin is loaded dynamically, use loadChildren
in your routing module.
TypeScript import { NgModule } from '@angular/core'; import { RouterModule, Routes } from '@angular/router'; const routes: Routes = [ { path: 'plugin', loadChildren: () => import('path/to/plugin').then(m => m.PluginModule) } ]; @NgModule({ imports: [RouterModule.forRoot(routes)], exports: [RouterModule] }) export class AppRoutingModule { } // Use code with caution. // Configuring the Plugin: Plugin Configuration: Provide necessary configuration to the plugin. TypeScript import { PluginConfig } from 'path/to/plugin'; @NgModule({ providers: [ { provide: PluginConfig, useValue: { apiKey: 'yourApiKey' } } ] }) export class AppModule { }
Isolating the main app from errors in a plugin
Error Handling in Plugins:
Try-Catch Blocks: Use try-catch blocks to manage exceptions within the plugin.
Custom Error Handling: Develop a custom mechanism for handling errors specific to the plugin.
Logging: Log errors to aid in debugging.
Error Isolation:
Plugin-Specific Error Handling: Address errors within the plugin to avoid impacting the core application.
Error Boundaries: Utilize Angular's error boundaries to prevent components from being affected by errors.
Observables and Error Handling: Implement appropriate error handling practices with Observables.
TypeScript import { Component, ErrorHandler } from '@angular/core'; @Component({ // ... }) export class AppComponent { constructor(private errorHandler: ErrorHandler) {} handleError(error: any) { // Custom error handling logic this.errorHandler.handleError(error); } }
Advanced Plugin Development
Server-side rendering and plugin architecture
Server-Side Rendering (SSR) and Plugins:
SSR can greatly improve plugin performance by providing pre-rendered HTML content, enhancing SEO, and decreasing the time to interactive. However, it introduces additional challenges in plugin development.
Considerations:
Plugin Compatibility: Verify that plugins are compatible with SSR environments.
Data Fetching: Plugins may need to retrieve data on the server-side.
State Management: Ensure consistent state management between the server and client.
Dynamic Content: Manage the generation of dynamic content within the plugin's SSR context.
Error Handling: Develop robust error handling mechanisms to address SSR failures.
TypeScript // Plugin component import { Component, OnInit } from '@angular/core'; import { Meta, Title } from '@angular/platform-browser'; @Component({ // ... }) export class PluginComponent implements OnInit { constructor(private meta: Meta, private title: Title) {} ngOnInit() { // Server-side data fetching or pre-rendering logic this.meta.addTag({ name: 'description', content: 'Plugin description' }); this.title.setTitle('Plugin Title'); } }
Best practices for developing and maintaining a plugin
Modularity and Reusability:
Decompose the plugin into smaller, self-contained components or modules. This approach improves maintainability, testability, and the possibility of reusing components in other projects.
Comprehensive Testing:
Adopt a thorough testing strategy that includes unit tests, integration tests, and end-to-end tests. Comprehensive testing helps ensure the plugin's reliability and avoids unexpected problems.
Clear and Consistent Documentation:
Offer detailed documentation that covers installation, configuration, usage, and API reference. Well-documented plugins are simpler to integrate and manage.
Performance Optimization:
Enhance the plugin's performance by reducing resource consumption, utilizing lazy loading, and considering server-side rendering when relevant.
Conclusion
In this article, we've explored the process of creating Angular modular plugins. We've examined the advantages, architecture, and implementation strategies for integrating plugins into Angular applications. By delving into the fundamental concepts, design, and implementation specifics, we've demonstrated how to build a robust and adaptable plugin system within an Angular framework.
This approach enables developers to create modular, maintainable, and expandable applications that can easily adapt to changing needs and incorporate external functionalities.
Please complete your information below to login.